There’s quite a bit to learn about search intent, from utilizing deep studying to deduce search intent by classifying textual content and breaking down SERP titles utilizing Pure Language Processing (NLP) methods, to clustering based mostly on semantic relevance, with the advantages defined.
Not solely do we all know the advantages of deciphering search intent, however we even have plenty of methods at our disposal for scale and automation.
So, why do we’d like one other article on automating search intent?
Search intent is ever extra vital now that AI search has arrived.
Whereas extra was typically within the 10 blue hyperlinks search period, the other is true with AI search know-how, as these platforms typically search to reduce the computing prices (per FLOP) in an effort to ship the service.
SERPs Nonetheless Include The Finest Insights For Search Intent
The methods to this point contain doing your personal AI, that’s, getting all the copy from titles of the rating content material for a given key phrase after which feeding it right into a neural community mannequin (which it’s important to then construct and take a look at) or utilizing NLP to cluster key phrases.
What should you don’t have time or the information to construct your personal AI or invoke the Open AI API?
Whereas cosine similarity has been touted as the reply to serving to web optimization professionals navigate the demarcation of subjects for taxonomy and website buildings, I nonetheless keep that search clustering by SERP outcomes is a far superior methodology.
That’s as a result of AI could be very eager to floor its outcomes on SERPs and for good motive – it’s modelled on person behaviors.
There may be one other manner that makes use of Google’s very personal AI to do the be just right for you, with out having to scrape all of the SERPs content material and construct an AI mannequin.
Let’s assume that Google ranks website URLs by the probability of the content material satisfying the person question in descending order. It follows that if the intent for 2 key phrases is identical, then the SERPs are prone to be related.
For years, many web optimization professionals in contrast SERP outcomes for key phrases to deduce shared (or shared) search intent to remain on prime of core updates, so that is nothing new.
The worth-add right here is the automation and scaling of this comparability, providing each pace and larger precision.
How To Cluster Key phrases By Search Intent At Scale Utilizing Python (With Code)
Assuming you’ve your SERPs ends in a CSV obtain, let’s import it into your Python pocket book.
1. Import The Record Into Your Python Pocket book
import pandas as pd
import numpy as np
serps_input = pd.read_csv('knowledge/sej_serps_input.csv')
del serps_input['Unnamed: 0']
serps_input
Beneath is the SERPs file now imported right into a Pandas dataframe.
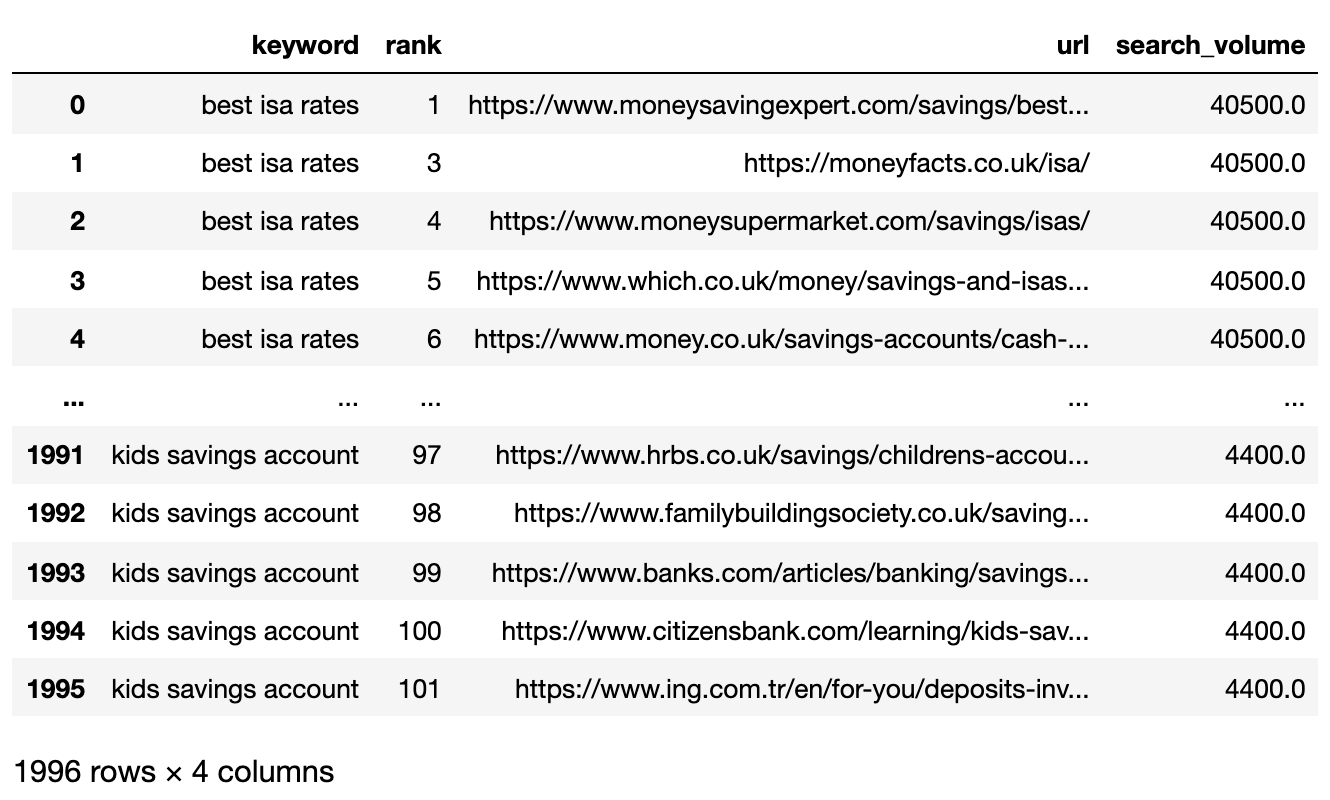
2. Filter Knowledge For Web page 1
We wish to evaluate the Web page 1 outcomes of every SERP between key phrases.
We’ll cut up the dataframe into mini key phrase dataframes to run the filtering perform earlier than recombining right into a single dataframe, as a result of we wish to filter on the key phrase degree:
# Break up
serps_grpby_keyword = serps_input.groupby("key phrase")
k_urls = 15
# Apply Mix
def filter_k_urls(group_df):
filtered_df = group_df.loc[group_df['url'].notnull()]
filtered_df = filtered_df.loc[filtered_df['rank']
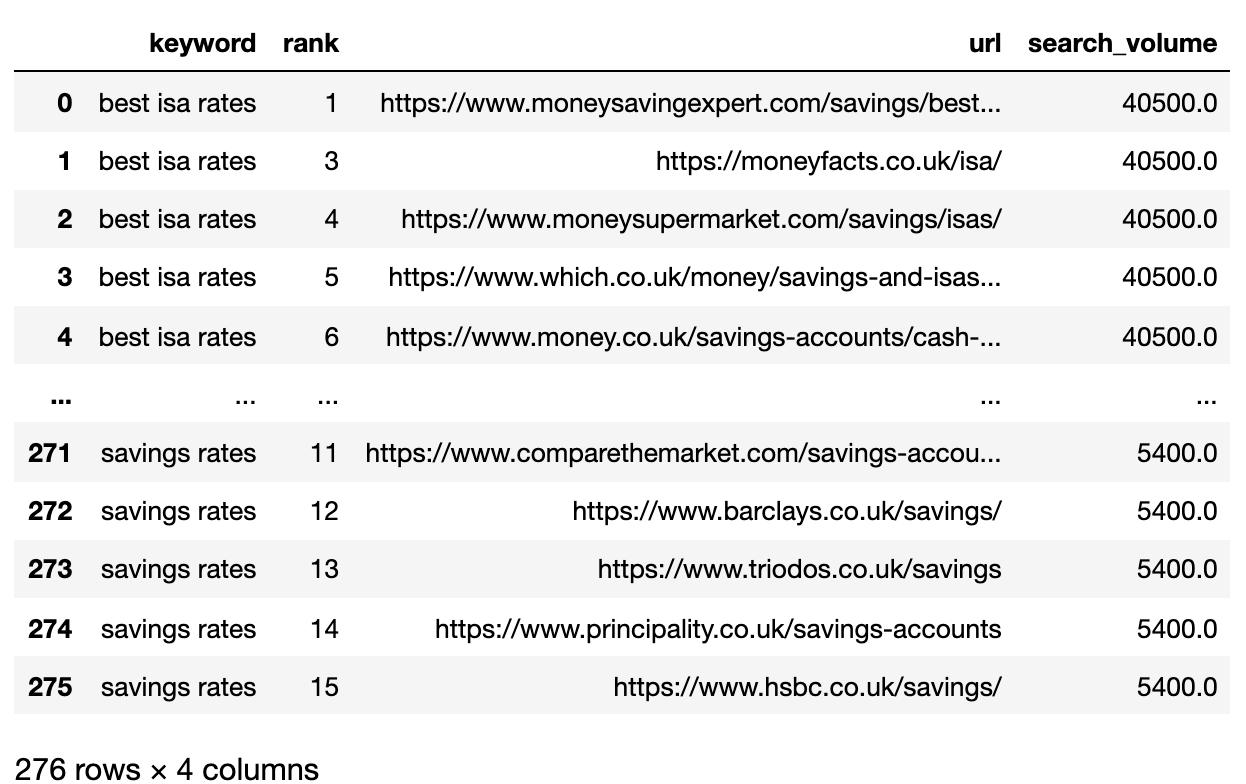
3. Convert Rating URLs To A String
As a result of there are extra SERP end result URLs than key phrases, we have to compress these URLs right into a single line to signify the key phrase’s SERP.
Right here’s how:
# convert outcomes to strings utilizing Break up Apply Mix
filtserps_grpby_keyword = filtered_serps_df.groupby("key phrase")
def string_serps(df):
df['serp_string'] = ''.be a part of(df['url'])
return df # Mix strung_serps = filtserps_grpby_keyword.apply(string_serps)
# Concatenate with preliminary knowledge body and clear
strung_serps = pd.concat([strung_serps],axis=0)
strung_serps = strung_serps[['keyword', 'serp_string']]#.head(30)
strung_serps = strung_serps.drop_duplicates()
strung_serps
Beneath reveals the SERP compressed right into a single line for every key phrase.
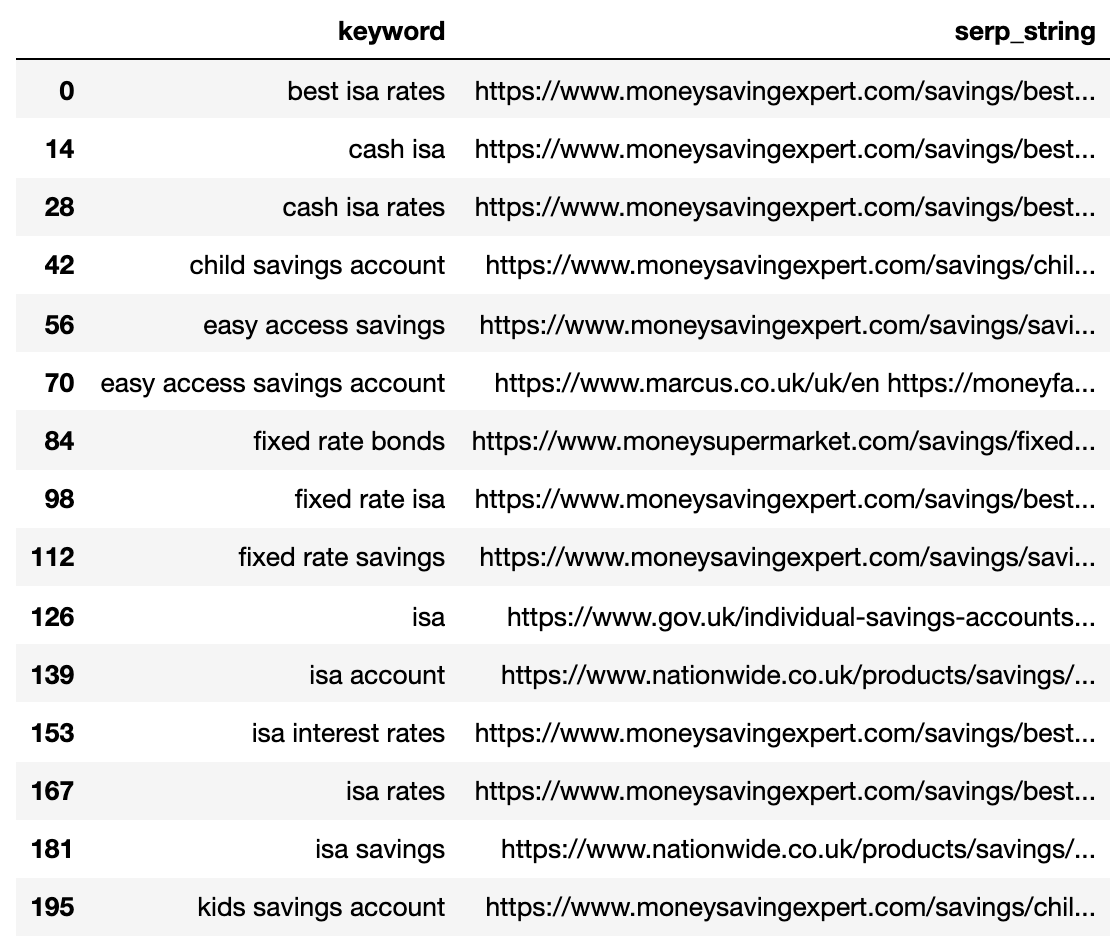
4. Evaluate SERP Distance
To carry out the comparability, we now want each mixture of key phrase SERP paired with different pairs:
# align serps
def serps_align(ok, df):
prime_df = df.loc[df.keyword == k]
prime_df = prime_df.rename(columns = {"serp_string" : "serp_string_a", 'key phrase': 'keyword_a'})
comp_df = df.loc[df.keyword != k].reset_index(drop=True)
prime_df = prime_df.loc[prime_df.index.repeat(len(comp_df.index))].reset_index(drop=True)
prime_df = pd.concat([prime_df, comp_df], axis=1)
prime_df = prime_df.rename(columns = {"serp_string" : "serp_string_b", 'key phrase': 'keyword_b', "serp_string_a" : "serp_string", 'keyword_a': 'key phrase'})
return prime_df
columns = ['keyword', 'serp_string', 'keyword_b', 'serp_string_b']
matched_serps = pd.DataFrame(columns=columns)
matched_serps = matched_serps.fillna(0)
queries = strung_serps.key phrase.to_list()
for q in queries:
temp_df = serps_align(q, strung_serps)
matched_serps = matched_serps.append(temp_df)
matched_serps
The above reveals all the key phrase SERP pair mixtures, making it prepared for SERP string comparability.
There isn’t any open-source library that compares checklist objects by order, so the perform has been written for you beneath.
The perform “serp_compare” compares the overlap of websites and the order of these websites between SERPs.
import py_stringmatching as sm
ws_tok = sm.WhitespaceTokenizer()
# Solely evaluate the highest k_urls outcomes
def serps_similarity(serps_str1, serps_str2, ok=15):
denom = ok+1
norm = sum([2*(1/i - 1.0/(denom)) for i in range(1, denom)])
#use to tokenize the URLs
ws_tok = sm.WhitespaceTokenizer()
#preserve solely first ok URLs
serps_1 = ws_tok.tokenize(serps_str1)[:k]
serps_2 = ws_tok.tokenize(serps_str2)[:k]
#get positions of matches
match = lambda a, b: [b.index(x)+1 if x in b else None for x in a]
#positions intersections of kind [(pos_1, pos_2), ...]
pos_intersections = [(i+1,j) for i,j in enumerate(match(serps_1, serps_2)) if j is not None]
pos_in1_not_in2 = [i+1 for i,j in enumerate(match(serps_1, serps_2)) if j is None]
pos_in2_not_in1 = [i+1 for i,j in enumerate(match(serps_2, serps_1)) if j is None]
a_sum = sum([abs(1/i -1/j) for i,j in pos_intersections])
b_sum = sum([abs(1/i -1/denom) for i in pos_in1_not_in2])
c_sum = sum([abs(1/i -1/denom) for i in pos_in2_not_in1])
intent_prime = a_sum + b_sum + c_sum
intent_dist = 1 - (intent_prime/norm)
return intent_dist
# Apply the perform
matched_serps['si_simi'] = matched_serps.apply(lambda x: serps_similarity(x.serp_string, x.serp_string_b), axis=1)
# That is what you get
matched_serps[['keyword', 'keyword_b', 'si_simi']]
Now that the comparisons have been executed, we will begin clustering key phrases.
We shall be treating any key phrases which have a weighted similarity of 40% or extra.
# group key phrases by search intent
simi_lim = 0.4
# be a part of search quantity
keysv_df = serps_input[['keyword', 'search_volume']].drop_duplicates()
keysv_df.head()
# append subject vols
keywords_crossed_vols = serps_compared.merge(keysv_df, on = 'key phrase', how = 'left')
keywords_crossed_vols = keywords_crossed_vols.rename(columns = {'key phrase': 'subject', 'keyword_b': 'key phrase',
'search_volume': 'topic_volume'})
# sim si_simi
keywords_crossed_vols.sort_values('topic_volume', ascending = False)
# strip NAN
keywords_filtered_nonnan = keywords_crossed_vols.dropna()
keywords_filtered_nonnan
We now have the potential subject identify, key phrases SERP similarity, and search volumes of every.
You’ll observe that key phrase and keyword_b have been renamed to subject and key phrase, respectively.
Now we’re going to iterate over the columns within the dataframe utilizing the lambda method.
The lambda method is an environment friendly option to iterate over rows in a Pandas dataframe as a result of it converts rows to an inventory versus the .iterrows() perform.
Right here goes:
queries_in_df = checklist(set(matched_serps['keyword'].to_list()))
topic_groups = {}
def dict_key(dicto, keyo):
return keyo in dicto
def dict_values(dicto, vala):
return any(vala in val for val in dicto.values())
def what_key(dicto, vala):
for ok, v in dicto.objects():
if vala in v:
return ok
def find_topics(si, keyw, topc):
if (si >= simi_lim):
if (not dict_key(sim_topic_groups, keyw)) and (not dict_key(sim_topic_groups, topc)):
if (not dict_values(sim_topic_groups, keyw)) and (not dict_values(sim_topic_groups, topc)):
sim_topic_groups[keyw] = [keyw]
sim_topic_groups[keyw] = [topc]
if dict_key(non_sim_topic_groups, keyw):
non_sim_topic_groups.pop(keyw)
if dict_key(non_sim_topic_groups, topc):
non_sim_topic_groups.pop(topc)
if (dict_values(sim_topic_groups, keyw)) and (not dict_values(sim_topic_groups, topc)):
d_key = what_key(sim_topic_groups, keyw)
sim_topic_groups[d_key].append(topc)
if dict_key(non_sim_topic_groups, keyw):
non_sim_topic_groups.pop(keyw)
if dict_key(non_sim_topic_groups, topc):
non_sim_topic_groups.pop(topc)
if (not dict_values(sim_topic_groups, keyw)) and (dict_values(sim_topic_groups, topc)):
d_key = what_key(sim_topic_groups, topc)
sim_topic_groups[d_key].append(keyw)
if dict_key(non_sim_topic_groups, keyw):
non_sim_topic_groups.pop(keyw)
if dict_key(non_sim_topic_groups, topc):
non_sim_topic_groups.pop(topc)
elif (keyw in sim_topic_groups) and (not topc in sim_topic_groups):
sim_topic_groups[keyw].append(topc)
sim_topic_groups[keyw].append(keyw)
if keyw in non_sim_topic_groups:
non_sim_topic_groups.pop(keyw)
if topc in non_sim_topic_groups:
non_sim_topic_groups.pop(topc)
elif (not keyw in sim_topic_groups) and (topc in sim_topic_groups):
sim_topic_groups[topc].append(keyw)
sim_topic_groups[topc].append(topc)
if keyw in non_sim_topic_groups:
non_sim_topic_groups.pop(keyw)
if topc in non_sim_topic_groups:
non_sim_topic_groups.pop(topc)
elif (keyw in sim_topic_groups) and (topc in sim_topic_groups):
if len(sim_topic_groups[keyw]) > len(sim_topic_groups[topc]):
sim_topic_groups[keyw].append(topc)
[sim_topic_groups[keyw].append(x) for x in sim_topic_groups.get(topc)]
sim_topic_groups.pop(topc)
elif len(sim_topic_groups[keyw])
Beneath reveals a dictionary containing all of the key phrases clustered by search intent into numbered teams:
{1: ['fixed rate isa',
'isa rates',
'isa interest rates',
'best isa rates',
'cash isa',
'cash isa rates'],
2: ['child savings account', 'kids savings account'],
3: ['savings account',
'savings account interest rate',
'savings rates',
'fixed rate savings',
'easy access savings',
'fixed rate bonds',
'online savings account',
'easy access savings account',
'savings accounts uk'],
4: ['isa account', 'isa', 'isa savings']}
Let’s stick that right into a dataframe:
topic_groups_lst = []
for ok, l in topic_groups_numbered.objects():
for v in l:
topic_groups_lst.append([k, v])
topic_groups_dictdf = pd.DataFrame(topic_groups_lst, columns=['topic_group_no', 'keyword'])
topic_groups_dictdf
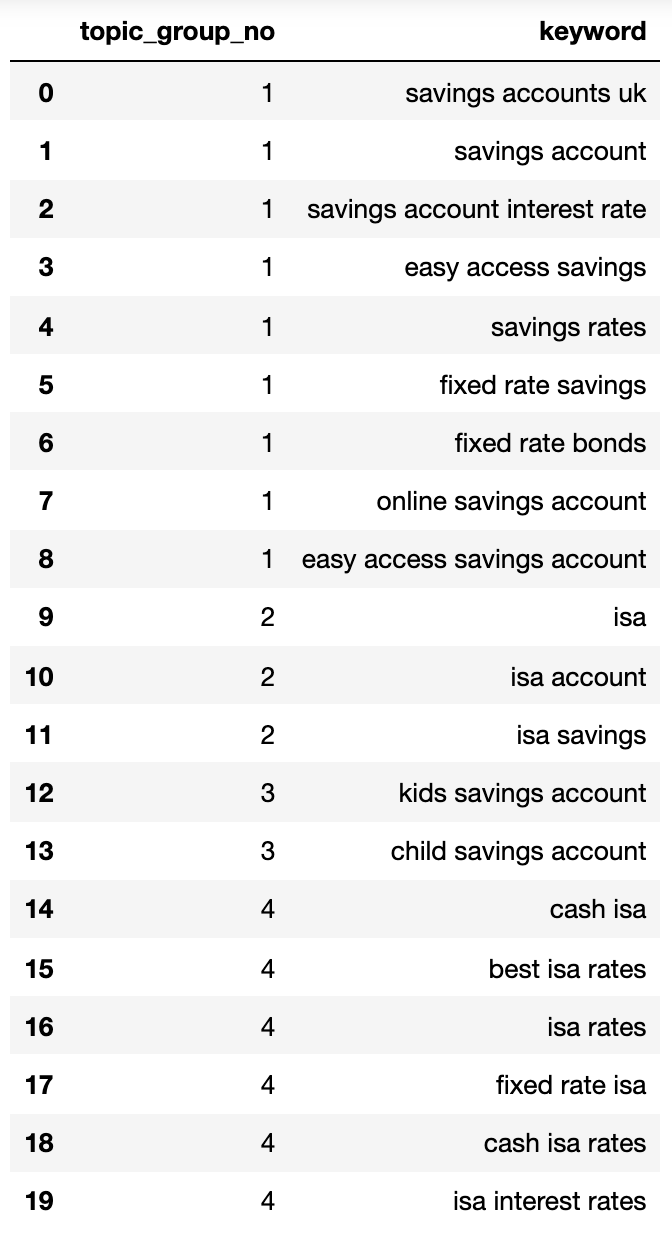
The search intent teams above present an excellent approximation of the key phrases inside them, one thing that an web optimization knowledgeable would seemingly obtain.
Though we solely used a small set of key phrases, the strategy can clearly be scaled to hundreds (if no more).
Activating The Outputs To Make Your Search Higher
In fact, the above may very well be taken additional utilizing neural networks, processing the rating content material for extra correct clusters and cluster group naming, as among the business merchandise on the market already do.
For now, with this output, you may:
- Incorporate this into your personal web optimization dashboard programs to make your tendencies and web optimization reporting extra significant.
- Construct higher paid search campaigns by structuring your Google Advertisements accounts by search intent for a better High quality Rating.
- Merge redundant side ecommerce search URLs.
- Construction a buying website’s taxonomy in keeping with search intent as a substitute of a typical product catalog.
I’m positive there are extra purposes that I haven’t talked about – be at liberty to touch upon any vital ones that I’ve not already talked about.
In any case, your web optimization key phrase analysis simply received that little bit extra scalable, correct, and faster!
Obtain the full code right here in your personal use.
Extra Sources:
Featured Picture: Buch and Bee/Shutterstock