Constructing functions with giant language fashions (LLMs) is thrilling, because it lets us create sensible, interactive techniques. Nevertheless, making these apps extra complicated brings alongside challenges, particularly when a number of LLMs work collectively. So, how can we handle the stream of knowledge between them? How can we be sure that they work easily and perceive the duty? LangGraph is the reply to all such questions. This free tutorial is an effective way for newcomers to know how LangGraph can resolve these issues. With hands-on examples and full code, this information will educate you tips on how to handle a number of LLMs successfully, making your functions extra highly effective and environment friendly.
Understanding LangGraph
LangGraph is a strong library, which is part of LangChain instruments. It helps streamline the combination of LLMs, making certain they work collectively seamlessly to know and execute duties. It presents a neat option to construct and deal with LLM apps with many brokers.
LangGraph lets builders arrange how a number of LLM brokers discuss to one another. It reveals these workflows as graphs with cycles. This helps in holding the communication clean and performing complicated duties nicely. LangGraph is greatest when utilizing Directed Acyclic Graphs (DAGs) for straight line duties. However since it’s cyclic and provides the flexibility to loop again, it permits for extra complicated and versatile techniques. It’s like how a sensible agent would possibly rethink issues and use new info to replace responses or change its selections.
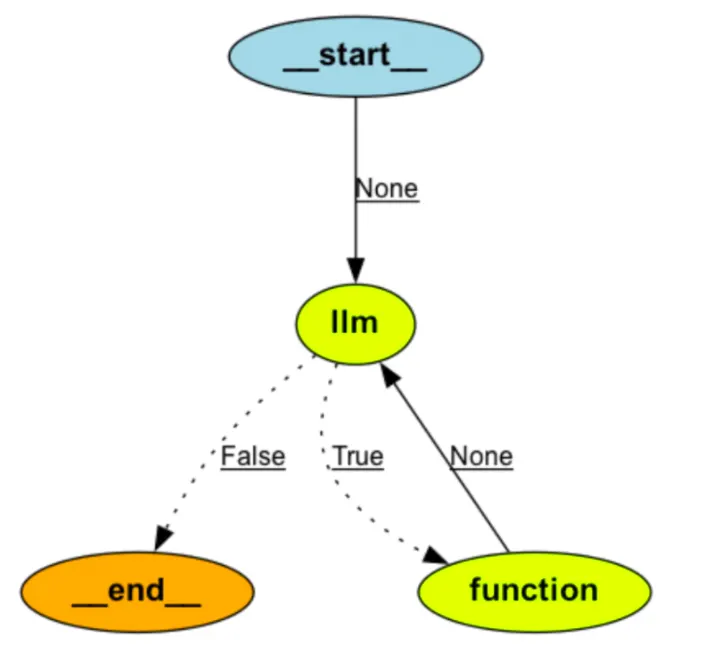
Additionally Learn: What’s LangGraph?
Key Ideas of LangGraph
Listed here are among the key ideas of LangGraph that it is advisable to know:
1. Graph Constructions
LangGraph’s core thought is utilizing a graph for the applying’s workflow. This graph has two foremost elements – nodes and edges.
- Nodes: Nodes are the elemental constructing blocks representing discrete models of labor or computation inside the workflow. Every node is a Python perform that processes the present state and returns an up to date state. Nodes can carry out duties akin to calling an LLM and interacting with instruments or APIs for manipulating knowledge.
- Edges: Edges join nodes and outline the stream of execution. They are often:
- Easy edges: Direct, unconditional transitions from one node to a different.
- Conditional edges: Branching logic that directs stream primarily based on node outputs, much like if-else statements. This permits dynamic decision-making inside the workflow.
2. State Administration
Protecting observe of what’s occurring is significant when you might have many brokers. All brokers have to know the present standing of the duty. LangGraph handles this by managing the state routinely. The library retains observe of and updates a foremost state object. It does this because the brokers do their jobs. The state object holds vital info. It’s obtainable at totally different factors within the workflow. This might embody the chat historical past.
In a chatbot, the state can save the dialog. This helps the bot reply utilizing what was stated earlier than. It may well additionally retailer context knowledge, like consumer likes, previous actions, and so on. or exterior knowledge. Brokers can use this for making selections. Inner variables will also be saved right here. Brokers would possibly use the state to trace flags, counts, or different values. These assist information their actions and choices.
3. Multi-agent Programs
A multi-agent system consists of a number of impartial brokers that work collectively or compete to attain a typical aim. These brokers use LLMs to make choices and management the stream of an utility. The complexity of a system can develop as extra brokers and duties are added. This will likely result in challenges like poor resolution making, context administration, and the necessity for specialization. A multi-agent system solves these issues by breaking the system into smaller brokers, every specializing in a particular process, akin to planning or analysis.
The principle advantages of utilizing a multi-agent system is modularity, specialization, and management. Modularity is for straightforward growth, testing and upkeep, whereas specialization ensures that professional brokers enhance general efficiency. Management ensures which you could clearly inform how the brokers ought to talk.
Additionally Learn: A Complete Information to Constructing Agentic RAG Programs with LangGraph
Architectures in Multi-agent Programs
Listed here are the varied varieties of architectures adopted in multi-agent techniques.
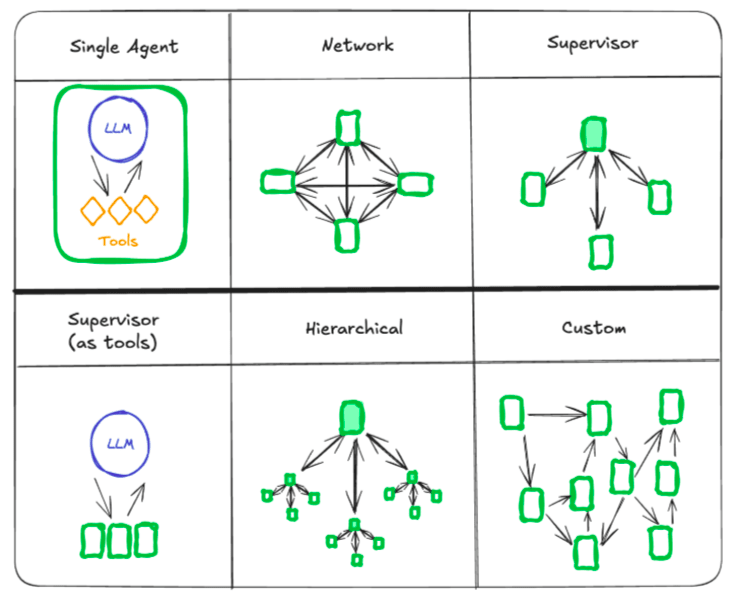
1. Community Structure: On this structure, each agent communicates with each different agent, and every can then resolve which agent they need to name subsequent. That is very useful when there isn’t any clear sequence of operations. Under is an easy instance of the way it works utilizing StateGraph.
from langchain_openai import ChatOpenAI
from langgraph.sorts import Command
from langgraph.graph import StateGraph
mannequin = ChatOpenAI()
def agent_1(state) -> Command:
response = mannequin.invoke(...)
return Command(goto=response["next_agent"], replace={"messages": [response["content"]]})
builder = StateGraph()
builder.add_node(agent_1)
builder.compile()
2. Supervisor Structure: A supervisor agent controls the choice making course of and routes duties to the suitable brokers. Right here’s a pattern of the way it’s finished:
def supervisor(state) -> Command:
response = mannequin.invoke(...)
return Command(goto=response["next_agent"])
builder = StateGraph()
builder.add_node(supervisor)
builder.compile()
3. Supervisor with Software-calling: On this structure, a supervisor agent makes use of a tool-calling agent to resolve which instrument (or agent) to make use of. The instrument executes duties and returns outcomes that information the subsequent management stream resolution.. A typical sample right here is to have a tool-wrapped perform:
def agent_1(state):
response = mannequin.invoke(...)
return response.content material
4. Hierarchical Structure: This method addresses the complexity of multi-agent techniques by organizing brokers into groups, every with its personal supervisor. The highest-level supervisor directs which staff to name. For example:
def top_level_supervisor(state):
response = mannequin.invoke(...)
return Command(goto=response["next_team"])
builder = StateGraph()
builder.add_node(top_level_supervisor)
builder.compile()
5. Handoffs in Multi-agent Programs: Handoffs enable one agent to cross management to a different, facilitating a stream from one agent to the subsequent. Every agent returns a Command object that specifies the subsequent agent to name and ship any updates to the state.
def agent(state) -> Command:
goto = get_next_agent(...)
return Command(goto=goto, replace={"my_state_key": "my_state_value"})
In complicated techniques, brokers could also be nested inside subgraphs, the place a node in a subgraph can direct management to a different agent outdoors its graph:
def some_node_inside_alice(state):
return Command(goto="bob", graph=Command.PARENT)
Multi-agent techniques allow modular and specialised designs the place brokers independently deal with duties and talk for environment friendly problem-solving. Architectures like community, supervisor, and hierarchical techniques every serve particular wants, whereas handoffs guarantee clean transitions between brokers, sustaining flexibility and management.
Do take a look at this free course to study extra about Constructing a Collaborative Multi-Agent System with LangGraph.
4. Persistence
Persistence means saving the progress of a course of with the intention to come again to it later, even after some interruptions. Every step’s state is saved, which helps with error restoration. It helps human suggestions throughout runs. You can too replay steps to debug or attempt new paths.
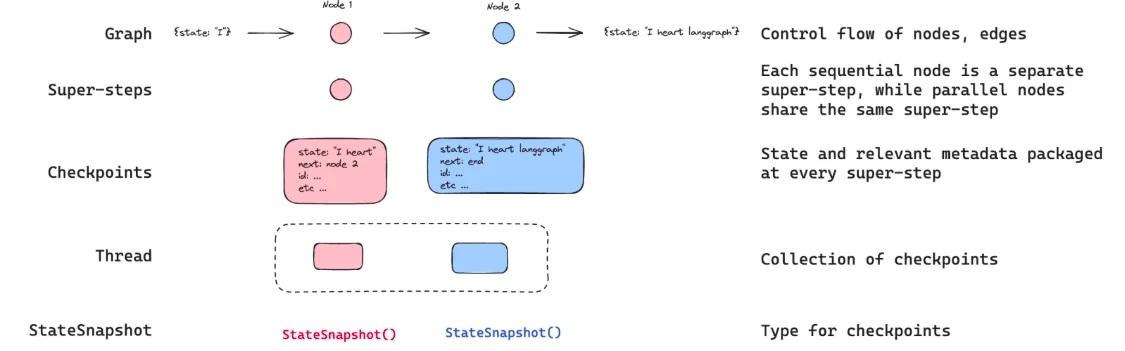
In LangGraph, persistence is finished utilizing checkpointers. Right here, the graph’s state is saved after each main step and every saved state is known as a checkpoint. All of the checkpoints are grouped inside a thread (the dialog historical past for a selected run).
Checkpointing is finished routinely and also you don’t at all times have to configure it manually. A checkpoint is sort of a snapshot of the graph’s state that features:
- config: Configuration information used throughout that step
- metadata: Step particulars (e.g., which node is working)
- values: The precise state values at that time
- subsequent: The subsequent node(s) that will probably be run
- duties: Information on what’s coming or errors
Every graph, whereas execution, wants a thread ID to group its checkpoints. You may present this thread id utilizing config: Under is a pattern of how it may be finished:
config = {"configurable": {"thread_id": "1"}}
To fetch the newest state inside a thread, use the beneath code:
graph.get_state({"configurable": {"thread_id": "1"}})
The beneath code reveals how one can get a particular checkpoint:
graph.get_state({
"configurable": {
"thread_id": "1",
"checkpoint_id": "your_checkpoint_id"
}
})
To get the state historical past or fetch all earlier states, use this code:
historical past = graph.get_state_history({"configurable": {"thread_id": "1"}})
You can too replace or edit the state manually at any level, utilizing:
graph.update_state(
config={"configurable": {"thread_id": "1"}},
values={"foo": "new_value"}
)
Additionally Learn: Tips on how to Construct a LangChain Chatbot with Reminiscence?
5. Human-in-the-Loop Integration
Human-in-the-loop helps you to add human suggestions at key steps of an automatic LangGraph workflow. That is essential in sure duties since LLMs might generate unsure or dangerous outputs akin to in instrument calls, content material era, or decision-making. LangGraph’s interrupt() perform makes this doable by pausing the graph, surfacing knowledge to a human, and resuming with their enter utilizing the Command(resume=worth) methodology. This allows evaluation, correction, or knowledge entry.
Human-in-the-loop helps patterns like Approve/Reject, Edit State, Present Enter, or Multi-turn Conversations. To make use of it, outline a checkpointer and add an interrupt() inside a node. You may resume the graph utilizing Command after human enter.
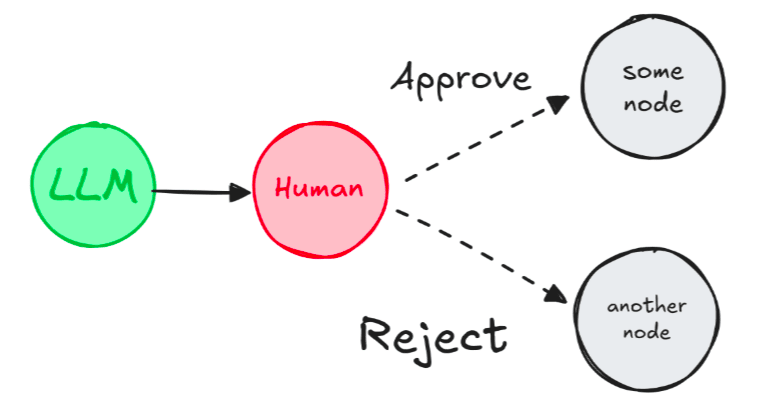
Under is a pattern of how you should use Human-in-the-loop in LangGraph.
from langgraph.sorts import interrupt, Command
def human_node(state):
worth = interrupt({"text_to_revise": state["some_text"]})
return {"some_text": worth}
graph = graph_builder.compile(checkpointer=checkpointer)
graph.invoke(some_input, config={"configurable": {"thread_id": "some_id"}})
graph.invoke(Command(resume="Edited textual content"), config={"configurable": {"thread_id": "some_id"}})
This retains workflows interactive, auditable, and correct excellent for high-stakes or collaborative AI use instances.
6. Streaming
LangGraph streams outputs as they’re created which lets customers see outcomes sooner. This improves their expertise with LLMs. Streaming helps you construct responsive apps by displaying you real-time progress. There are 3 foremost knowledge sorts to stream: workflow progress, LLM tokens, and customized updates.
Use .stream() (sync) or .astream() (async) to stream outputs. You may set stream_mode to regulate what you get:
- “values”: full state after every graph step
- “updates”: modifications solely after every node
- “customized”: any customized knowledge you log in a node
- “messages”: LLM token stream with metadata
- “debug”: all information all through the run
You may cross a number of modes like this:
for stream_type, knowledge in graph.stream(inputs, stream_mode=["updates", "messages"]):
if stream_type == "messages":
print(knowledge[0].content material) # AIMessageChunk
elif stream_type == "updates":
print(knowledge) # State replace
Use .astream_events() if you’d like a full occasion stream. That is excellent when migrating massive apps.
Professional tip: For real-time UI suggestions, use “messages” for token-wise streaming and “updates” for backend state.
Why Use LangGraph?
LangGraph is right for builders constructing sensible and versatile AI brokers. Right here’s why:
- Dependable and controllable: Add moderation checks and human approvals. It retains context alive for lengthy duties.
- Customized and extensible: Use low-level instruments to construct brokers your approach. Design techniques with brokers that every play a particular function.
- Nice streaming: See every token and step reside, monitoring agent considering because it occurs.
You can too take this course from the Langchain academy to study extra.
Constructing the Easiest Graph
Now that we’ve got seen the important thing parts of LangGraph, let’s attempt to construct a primary graph with three nodes and one conditional edge. This easy instance reveals tips on how to invoke a graph involving the important thing ideas of State, Nodes, and Edges.
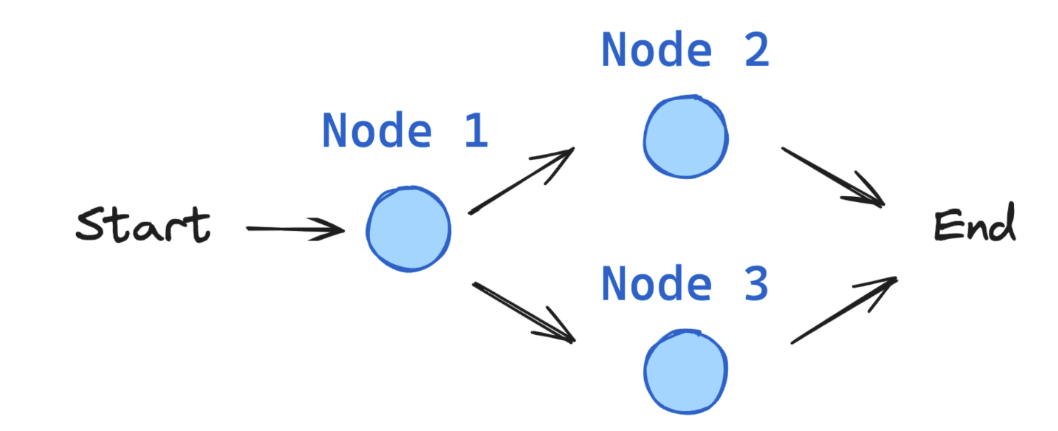
Step 1: Outline the Graph State
The State defines the info construction which is shared between nodes. It acts like a shared reminiscence that flows by means of the graph.
from typing_extensions import TypedDict
class State(TypedDict):
graph_state: str
Right here, we’ve got used python’s TypeDict to declare that our state can have a single key referred to as the graph_state, which shops a string.
Step 2: Create the Nodes
Nodes are simply easy Python capabilities. Every one takes within the present state, modifies it, and returns the up to date state.
def node_1(state):
print("---Node 1---")
return {"graph_state": state['graph_state'] + " I'm"}
This perform appends “I’m” to no matter string is in graph_state.
def node_2(state):
print("---Node 2---")
return {"graph_state": state['graph_state'] + " extraordinarily blissful!"}
def node_3(state):
print("---Node 3---")
return {"graph_state": state['graph_state'] + " extraordinarily unhappy!"}
Right here, these two nodes add an emotional tone of “blissful!” or “unhappy!” to the sentence.
Step 3: Add Conditional Logic
Generally you need dynamic habits, the place the subsequent step depends upon logic or randomness. That’s what conditional edges allow.
import random
from typing import Literal
def decide_mood(state) -> Literal["node_2", "node_3"]:
if random.random()
This perform randomly picks between node_2 and node_3 with equal chance, simulating a easy temper selector.
Step 4: Assemble the Graph
Let’s convey all of it collectively utilizing LangGraph’s StateGraph class. That is the place we outline the complete graph construction.
from IPython.show import Picture, show
from langgraph.graph import StateGraph, START, END
# Initialize the graph with the state schema
builder = StateGraph(State)
# Add nodes to the graph
builder.add_node("node_1", node_1)
builder.add_node("node_2", node_2)
builder.add_node("node_3", node_3)
We begin with the START node and path to node_1. Then, we add a conditional edge from node_1 utilizing decide_mood. After that, the graph continues to both node_2 or node_3 and ends on the END node.
# Add edges to outline stream
builder.add_edge(START, "node_1")
builder.add_conditional_edges("node_1", decide_mood)
builder.add_edge("node_2", END)
builder.add_edge("node_3", END)
# Compile and visualize the graph
graph = builder.compile()
show(Picture(graph.get_graph().draw_mermaid_png()))
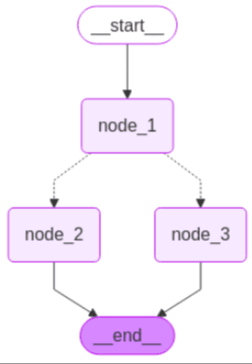
The compile() methodology performs primary validation, and draw_mermaid_png() helps you to visualize the graph as a Mermaid diagram.
Step 5: Invoke the Graph
Lastly, we will run the graph utilizing the invoke() methodology.
graph.invoke({"graph_state" : "Hello, that is Janvi."})
This begins the graph on the START node and initializes graph_state with the sentence “Hello, that is Janvi.”.
- node_1 appends ” I’m” → “Hello, that is Janvi.”
- decide_mood randomly chooses the trail
- node_2 or node_3 appends both ” extraordinarily blissful!” or ” extraordinarily unhappy!”
Output:

This output reveals how state flows and updates by means of every step of the graph.
Constructing a Help Chatbot with LangGraph Utilizing OpenAI
Now that we’ve got constructed the best graph within the above part, on this part, I’ll present you tips on how to use LangGraph to construct a help chatbot, beginning with primary performance and progressively including options like internet search, reminiscence, and human-in-loop. Alongside the best way, we are going to see the core LangGraph ideas as nicely.
Our aim right here is to create a chatbot that may reply questions utilizing internet search, bear in mind previous conversations, ask a human for assist when wanted, use a customized state for habits, and rewind dialog paths (enabled by checkpointing).
Additionally Learn: Construct an AI Coding Agent with LangGraph by LangChain
Setup
Earlier than constructing the chatbot, let’s set up the mandatory packages.
!pip set up -U langgraph langchain openai
This command installs:
- LangGraph: For constructing the graph construction.
- LangChain: For interacting with OpenAI’s language fashions.
- OpenAI: For utilizing OpenAI’s fashions (like GPT-4).
We have to securely present the OpenAI API key so the applying can authenticate and use the GPT fashions. This perform prompts for the important thing if it’s not already set within the surroundings.
import getpass
import os
def _set_env(var: str):
if not os.environ.get(var):
os.environ[var] = getpass.getpass(f"{var}: ")
_set_env("OPENAI_API_KEY")
Half 1: Construct a Primary Chatbot
We’ll begin by creating the best type of the chatbot.
1. Outline State
The state defines the info construction that will get handed between nodes within the graph. Right here, we outline a state with a single key, messages, which is able to maintain the listing of dialog messages.
from typing import Annotated
from typing_extensions import TypedDict
from langgraph.graph import StateGraph, START, END
from langgraph.graph.messae import add_messages
class State(TypedDict):
# 'messages' holds the listing of chat messages.
# 'add_messages' ensures new messages are added, not changed.
messages: Annotated[list, add_messages]
2. Create Graph Builder
The StateGraph object is the entry level for outlining the graph construction. It’s initialized with the State definition we simply created.
graph_builder = StateGraph(State)
3. Add Chatbot Node
We outline a Python perform chatbot that takes the present state, invokes OpenAI’s GPT mannequin with the messages from the state, and returns the LLM’s response as an replace to the messages key within the state.
import openai
# Initialize OpenAI GPT mannequin
openai.api_key = os.environ["OPENAI_API_KEY"]
def chatbot(state: State):
response = openai.Completion.create(
mannequin="gpt-4", # You can too use "gpt-3.5-turbo" or another OpenAI mannequin
immediate=state["messages"],
max_tokens=150
)
return {"messages": [response.choices[0].textual content.strip()]}
graph_builder.add_node("chatbot", chatbot)
4. Set Entry and Exit Factors
Outline the entry level (START) and exit level (END) for the graph execution.
graph_builder.add_edge(START, "chatbot")
graph_builder.add_edge("chatbot", END)
5. Compile the Graph
As soon as all nodes and edges are outlined, compile the graph construction.
graph = graph_builder.compile()
6. Visualize (Non-obligatory)
LangGraph permits visualizing the compiled graph construction. This helps perceive the stream of execution. We are able to visualize the graph utilizing instruments like pygraphviz or mermaid.
from IPython.show import Picture, show
attempt:
show(Picture(graph.get_graph().draw_mermaid_png()))
besides Exception:
cross # Non-obligatory visualization
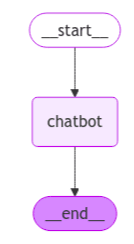
7. Run the Chatbot
Arrange a loop to work together with the chatbot. It takes consumer enter, packages it into the anticipated State format ({“messages”: […]}), and makes use of graph.stream to execute the graph. The stream methodology returns occasions because the graph progresses, and we print the assistant’s closing message.
def stream_graph_updates(user_input: str):
for occasion in graph.stream({"messages": [{"role": "user", "content": user_input}]}):
for worth in occasion.values():
print("Assistant:", worth["messages"][-1].content material)
# Loop to speak with the bot
whereas True:
attempt:
user_input = enter("Consumer: ")
if user_input.decrease() in ["quit", "exit", "q"]:
print("Goodbye!")
break
stream_graph_updates(user_input)
besides: # Fallback for environments with out enter()
user_input = "What have you learnt about LangGraph?"
print("Consumer: " + user_input)
stream_graph_updates(user_input)
break

Half 2: Enhancing the Chatbot with Instruments
To make the chatbot extra educated, particularly about current info, we’ll combine an internet search instrument (Tavily). This entails enabling the LLM to request instrument utilization and including graph parts to deal with the execution of those instruments.
1. Set up Software Necessities
Set up the mandatory library for the Tavily search instrument.
%pip set up -U tavily-python langchain_community
2. Set Software API Key
Configure the API key for the Tavily service.
_set_env("TAVILY_API_KEY") # Makes use of the perform outlined earlier
3. Outline the Software
Instantiate the TavilySearchResults instrument, which is able to return 2 outcomes. This instrument will probably be utilized by each the LLM and the graph.
from langchain_community.instruments.tavily_search import TavilySearchResults
# Create a Tavily search instrument occasion, limiting to 2 outcomes
instrument = TavilySearchResults(max_results=2)
instruments = [tool] # Record of instruments the bot can use
Half 3: Add Reminiscence to the Chatbot
To allow multi-turn conversations the place the bot remembers earlier messages, we introduce LangGraph’s checkpointing function.
Add Checkpointer
Use the MemorySaver checkpointer to retailer the dialog state in reminiscence. For manufacturing, you would possibly use a persistent backend like SQLite or Postgres.
from langgraph.checkpoint.reminiscence import MemorySaver
reminiscence = MemorySaver()
Half 4: Human-in-the-loop
Generally, the AI agent would possibly want human enter earlier than continuing. We obtain this by making a instrument that pauses the graph’s stream.
Outline Human Help Software
from langchain_core.instruments import instrument
from langgraph.sorts import interrupt
@instrument
def human_assistance(question: str) -> str:
print(f"Pausing for human help relating to: {question}")
# interrupt pauses graph execution and waits for enter
human_response = interrupt({"question": question})
return human_response["data"]
This instrument pauses the graph and waits for human enter earlier than continuing.
Deploying Your LangGraph Purposes
After getting constructed your LangGraph utility, the subsequent factor which it is advisable to do is working the app both in your native machine or cloud platforms for additional growth and testing. LangGraph gives us with a number of deployment choices which may have totally different workflows and infrastructure.
For deployment, LangGraph helps a number of choices. The Cloud SaaS mannequin handles every little thing for you. The Self-Hosted Knowledge Airplane helps you to run apps in your personal cloud whereas utilizing LangChain’s management airplane. With the Self-Hosted Management Airplane, you handle every little thing your self. Or go together with Standalone Containers for full flexibility utilizing Docker.
Use Circumstances of LangGraph
LangGraph is used to construct interactive and clever AI Brokers. Let’s discover and see a few of its use instances.
1. Improved Buyer Service: LangGraph is able to growing superior chatbots for buyer help. These chatbots are capable of recall previous purchases and buyer preferences.With the recalled previous they will reply to the queries in regards to the order and might hyperlink to people when vital. With this the shopper’s downside will be solved sooner.
2. Analysis Assistant for AI: A analysis assistant will also be created utilizing LangGraph. It may well search for scholarly articles after which spotlight vital info. The assistant can then extract the knowledge and this info then can be utilized by researchers and college students to achieve extra insights from varied fields.
3. Personalised Studying: With LangGraph we will additionally construct personalised or custom-made studying techniques which is able to alter the content material primarily based on the learner. This can assist the learner perceive the weaker space after which suggest assets primarily based on that. This creates a personalised studying expertise, bettering engagement and outcomes.
4. Streamlining Enterprise Duties: LangGraph may also assist us in automating enterprise processes. With this doc approval and mission administration will be automated and likewise the agent will also be used to research knowledge. Automation helps in rising productiveness and reduces human error, permitting groups to give attention to higher-level duties.
Study Extra: Dynamic AI Workflows By LangGraph ReAct Operate Calling
Conclusion
On this LangGraph tutorial for newcomers, you discovered tips on how to construct interactive AI techniques. These techniques transcend easy Q&A bots. By LangGraph examples, we noticed how LangGraph manages state, integrates a number of brokers, and permits human enter. The information confirmed tips on how to construct a help chatbot that may deal with internet searches, bear in mind previous interactions, and even contain human intervention.
The LangGraph tutorial for newcomers is superb for builders. It helps create highly effective, AI-driven functions. Through the use of LangGraph, we will construct versatile, adaptive techniques that may deal with complicated duties. Whether or not you’re constructing a chatbot, analysis assistant, or personalised studying instrument, LangGraph has the construction and instruments you want for environment friendly growth.
Steadily Requested Questions
A. LangGraph is a strong library that enables builders to make complicated and superior AI brokers which may work together with giant language fashions. It additionally helps in managing workflow utilizing graph construction. With the assistance of this graph construction a number of brokers will be constructed to deal with complicated duties.
A. LangGraph works by defining workflows as graphs. The graph consists of nodes (duties or computations) and edges (connections between duties). It handles state administration, ensuring every agent has the knowledge it must carry out its process and work together with different brokers.
A. LangGraph presents:
– State administration which retains observe of knowledge because the agent performs duties.
– Multi-agent help which permits a number of brokers to work collectively inside a graph.
– Persistence with checkpointers because it saves the state at every step which allow error restoration and debudding.
– Human-in-the-loop which helps in pausing the workflow for human evaluation and approval.
A. Sure, LangGraph will be very simply built-in with OpenAI’s GPT fashions. It permits us to construct functions that use the facility of LLMs, akin to chatbots and AI assistants, whereas managing complicated workflows and state throughout a number of brokers.
A. Sure, this LangGraph tutorial for newcomers is designed that will help you get began. It walks by means of key ideas with LangGraph examples and explains tips on how to construct techniques step-by-step. Moreover, the LangGraph tutorial for newcomers free gives assets for studying the framework for free of charge.
Login to proceed studying and revel in expert-curated content material.